LINQ to SQL provides a runtime infrastructure for managing relational data as objects without losing the ability to query. Your application is free to manipulate the objects while LINQ to SQL stays in the background tracking your changes automatically.It is possible to migrate current ADO.NET solutions to LINQ to SQL in a piecemeal fashion (sharing the same connections and transactions) since LINQ to SQL is simply another component in the ADO.NET family. LINQ to SQL also has extensive support for stored procedures, allowing reuse of the existing enterprise assets. In addition it can filter data in .NET end which are coming from the stored proc.
Following are the steps describe you how LINQ to Stored proc works together.
Step 1-First create a table in your SQL server database-
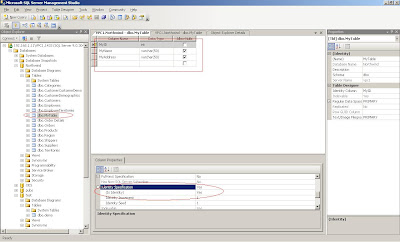
I have created a table MyTable in the Northwind database.The table contains three feilds(MyID int,MyName Varchar,MyAddress varchar) MyID is a autogenerate number and primary key in this table.
Put some data in yout table
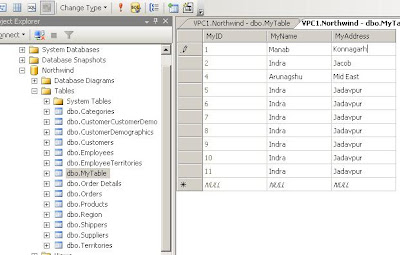
Step 2.Write the following stored proc in your database
-----------------------------------------------------------------------------------------------------------------------
USE [Northwind]
GO
/****** Object: StoredProcedure [dbo].[spMyTableDelete] Script Date: 12/07/2009 00:08:17 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[spMyTableDelete]
(
@MyID int
-- @MyName varchar(50),
-- @MyAddress varchar(50)
)
AS
BEGIN
SET NOCOUNT ON;
DELETE from dbo.MyTable where MyID=@MyID
--SELECT MyID,MyName,MyAddress from dbo.MyTable
END
--select * from MyTable
-------------------------------------------------------------------------------------------------------------------------
USE [Northwind]
GO
/****** Object: StoredProcedure [dbo].[spMyTableInsert] Script Date: 12/07/2009 00:09:25 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[spMyTableInsert]
(
@MyName varchar(50),
@MyAddress varchar(50)
)
AS
BEGIN
SET NOCOUNT ON;
INSERT INTO dbo.MyTable(MyName,MyAddress)VALUES(@MyName,@MyAddress)
END
------------------------------------------------------------------------------------------------------------------------
USE [Northwind]
GO
/****** Object: StoredProcedure [dbo].[spMyTableSelect] Script Date: 12/07/2009 00:10:08 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[spMyTableSelect]
-- (
-- @MyID int,
-- @MyName varchar(50),
-- @MyAddress varchar(50)
-- )
AS
BEGIN
SET NOCOUNT ON;
SELECT MyID,MyName,MyAddress from dbo.MyTable
END
--select * from MyTable
----------------------------------------------------------------------------------------------------------------------------
USE [Northwind]
GO
/****** Object: StoredProcedure [dbo].[spMyTableSelectMulti] Script Date: 12/07/2009 00:10:40 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[spMyTableSelectMulti]
-- (
-- @MyID int
-- @MyName varchar(50),
-- @MyAddress varchar(50)
-- )
AS
BEGIN
SET NOCOUNT ON;
SELECT MyID,MyName,MyAddress from dbo.MyTable where MyID=2
SELECT MyID,MyName,MyAddress from dbo.MyTable where MyID=1
END
--select * from MyTable
----------------------------------------------------------------------------------------------------------------------
USE [Northwind]
GO
/****** Object: StoredProcedure [dbo].[spMyTableSelectMultiWithOutputParam] Script Date: 12/07/2009 00:11:27 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[spMyTableSelectMultiWithOutputParam]
(
@MyID int,
@MyName varchar(50) output
-- @MyAddress varchar(50)
)
AS
BEGIN
SET NOCOUNT ON;
SELECT MyID,MyName,MyAddress from dbo.MyTable
SELECT @MyName=(SELECT MyName from dbo.MyTable where MyID=@MyID)
END
--select * from MyTable
------------------------------------------------------------------------------------------------------------------------------
USE [Northwind]
GO
/****** Object: StoredProcedure [dbo].[spMyTableUpdate] Script Date: 12/07/2009 00:12:25 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE PROCEDURE [dbo].[spMyTableUpdate]
(
@MyID int,
@MyName varchar(50),
@MyAddress varchar(50)
)
AS
BEGIN
SET NOCOUNT ON;
UPDATE dbo.MyTable SET MyName=@MyName,MyAddress=@MyAddress
WHERE MyID=@MyID
END
Step 3.Create a new web site in .NET IDE
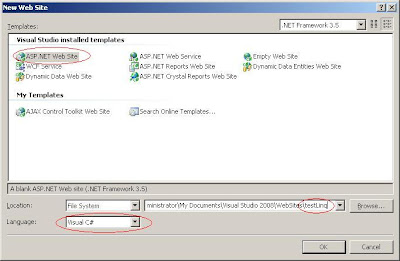
Step 4.Choose "Add New Item" on your App_Code folder
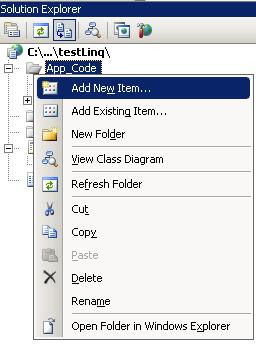
Step 5.Choose "LINQ to SQL Classes" in yourAdd New Item Dialog and put the file name "MyDataClasses.dbml".
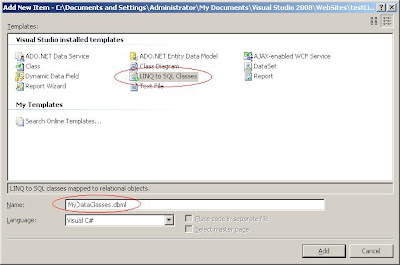
Step 6.Open the MyDataClasses.dbml and modify the name "MyDataContext".Click on "Server Explorer" in MyDataClasses.dbml
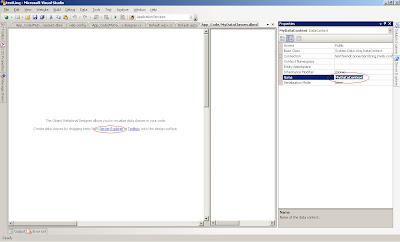
Step7.In the Server Explorer click on "Connect to database" icon
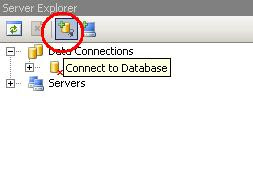
Step 8.In "Add Connection" dialog put the server name,database name and required credentials
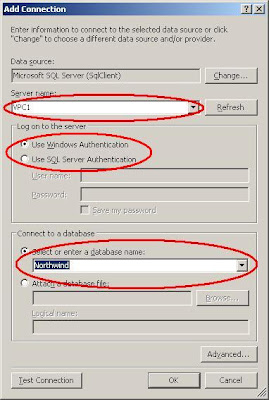
Step 9.Drag the requird Stored proc in your Method Pane
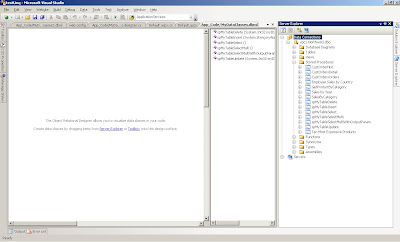
Step 10.If the Method Pane is not visible,right click on the designer and click on "Show Methods Pane".
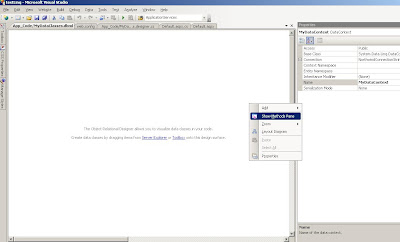
Step 11.Now go to your Default.aspx page and create few buttons ,event and a gridview
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="grdData" runat="server">
</asp:GridView>
</div>
<asp:Button ID="btnInsert" runat="server" OnClick="btnInsert_Click" Text="Insert" />
<asp:Button ID="btnUpdate" runat="server" OnClick="btnUpdate_Click" Text="Update" />
<asp:Button ID="btnSelect" runat="server" OnClick="btnSelect_Click" Text="Select" />
<asp:Button ID="btnDelete" runat="server" OnClick="btnDelete_Click" Text="Delete" />
<asp:Button ID="btnSelectMulti" runat="server" OnClick="btnSelectMulti_Click" Text="SelectMultipleDatatable" />
<asp:Button ID="btnSelectMultiWithOutputParam" runat="server" OnClick="btnSelectMultiWithOutputParam_Click"
Text="SelectMultipleWithOutputParameter" />
</form>
</body>
</html>
Step 12.In the Default.aspx.cs call the required stored procedure for Insert ,update ,select and Delete
MyDataContext db = new MyDataContext();
protected void btnInsert_Click(object sender, EventArgs e)
{
var q3 = db.spMyTableInsert("Indra", "Jadavpur");
}
protected void btnUpdate_Click(object sender, EventArgs e)
{
var update = db.spMyTableUpdate(2,"Indra", "Jadavpur");
}
protected void btnSelect_Click(object sender, EventArgs e)
{
var Select2 = from o in db.spMyTableSelect()
where o.MyID <= 12 select new { o.MyID, o.MyName, o.MyAddress };
grdData.DataSource = Select2;
grdData.DataBind();
} protected void btnDelete_Click(object sender, EventArgs e)
{
var delete = db.spMyTableDelete(3);
}
Step 13.Now if you like to call a stored proc like dbo.spMyTableSelectMultiWithOutputParam which has a output param write teh follwoing code in your btnSelectMultiWithOutputParam click event
protected void btnSelectMultiWithOutputParam_Click(object sender, EventArgs e)
{
string strCustName = "";
var q2 = db.spMyTableSelectMultiWithOutputParam(1, ref strCustName);
Response.Write(strCustName.ToString());
grdData.DataSource = q2; grdData.DataBind();
}
The spMyTableSelectMultiWithOutputParam is like this
ALTER PROCEDURE dbo.spMyTableSelectMultiWithOutputParam
(
@MyID int, @MyName varchar(50) output
) AS
BEGIN
SET NOCOUNT ON;
SELECT MyID,MyName,MyAddress from dbo.MyTable
SELECT @MyName=(SELECT MyName from dbo.MyTable where MyID=@MyID)
END Step
14.Now if you want to call a storedproc which is returning multiple select statement like spMyTableSelectMulti,do the following steps---
The stored proc is like this
ALTER PROCEDURE dbo.spMyTableSelectMulti
AS
BEGIN
SET NOCOUNT ON;
SELECT MyID,MyName,MyAddress from dbo.MyTable where MyID=2
SELECT MyID,MyName,MyAddress from dbo.MyTable where MyID=1
END
Open the MyDataClassesDesigner.cs and comment the class spMyTableSelectMultiResult .
Then create a new class file clsMultiRecords.cs in App_code and create two partial class spMyTableSelectMultiResult and spMyTableSelectMultiResult2 First Class return the first SQL query and the second one return the second sql query.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.Linq;
using System.Data.Linq.Mapping;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
/// <summary>
/// Summary description for clsMultiRecords
/// </summary>
public class clsMultiRecords
{
public clsMultiRecords()
{
//
// TODO: Add constructor logic here
//
}
//[Function(Name = "dbo.spMyTableSelectMulti"), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult)), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult2))]
//public IMultipleResults spMyTableSelectMulti()
//{
// IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
// return ((IMultipleResults)(result.ReturnValue));
//}
public partial class spMyTableSelectMultiResult
{
private int _MyID;
private string _MyName;
private string _MyAddress;
public spMyTableSelectMultiResult()
{
}
[Column(Storage = "_MyID", DbType = "Int NOT NULL")]
public int MyID
{
get
{
return this._MyID;
}
set
{
if ((this._MyID != value))
{
this._MyID = value;
}
}
}
[Column(Storage = "_MyName", DbType = "VarChar(50)")]
public string MyName
{
get
{
return this._MyName;
}
set
{
if ((this._MyName != value))
{
this._MyName = value;
}
}
}
[Column(Storage = "_MyAddress", DbType = "VarChar(50)")]
public string MyAddress
{
get
{
return this._MyAddress;
}
set
{
if ((this._MyAddress != value))
{
this._MyAddress = value;
}
}
}
}
public partial class spMyTableSelectMultiResult2
{
private int _MyID;
private string _MyName;
private string _MyAddress;
public spMyTableSelectMultiResult2()
{
}
[Column(Storage = "_MyID", DbType = "Int NOT NULL")]
public int MyID
{
get
{
return this._MyID;
}
set
{
if ((this._MyID != value))
{
this._MyID = value;
}
}
}
[Column(Storage = "_MyName", DbType = "VarChar(50)")]
public string MyName
{
get
{
return this._MyName;
}
set
{
if ((this._MyName != value))
{
this._MyName = value;
}
}
}
[Column(Storage = "_MyAddress", DbType = "VarChar(50)")]
public string MyAddress
{
get
{
return this._MyAddress;
}
set
{
if ((this._MyAddress != value))
{
this._MyAddress = value;
}
}
}
}
}
Again open the DataClassesDesigner.cs and delete the follwoing portion
[Function(Name = "dbo.spMyTableSelectMulti")]
public ISingleResult
spMyTableSelectMulti()
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
return ((ISingleResult)(result.ReturnValue));
}
and write the following code in place of that
[Function(Name = "dbo.spMyTableSelectMulti"), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult)), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult2))]
public IMultipleResults spMyTableSelectMulti()
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
return ((IMultipleResults)(result.ReturnValue));
}
This method will return the IMultipleResults instead of ISingleResult. Mind it,if you open the dbml(MyDataClasses.dbml not MyDataClasses.designer.cs) file and save/modify it, this above portion will be overridden by ISingleResult. Now go to the click event of btnSelectMulti and write the following code
protected void btnSelectMulti_Click(object sender, EventArgs e)
{
IMultipleResults results = new MyDataContext().spMyTableSelectMulti(); List result1 = results.GetResult().ToList();
List result2 = results.GetResult().ToList();
grdData.DataSource = result2;
grdData.DataBind();
}
Step 15.Now run your application ,you can now see the following output,click on different button to obeserve the operation
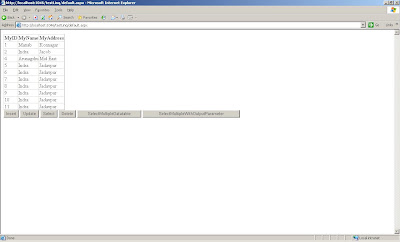
I am attaching the complete code for Default.aspx.cs ,MyDataContext class and clsMultiRecords.cs
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Collections;
using System.Data.Linq;
public partial class _Default : System.Web.UI.Page
{
MyDataContext db = new MyDataContext();
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnInsert_Click(object sender, EventArgs e)
{
var q3 = db.spMyTableInsert("Indra", "Jadavpur");
}
protected void btnUpdate_Click(object sender, EventArgs e)
{
var update = db.spMyTableUpdate(2,"Indra", "Jadavpur");
}
protected void btnSelect_Click(object sender, EventArgs e)
{
var Select2 = from o in db.spMyTableSelect()
where o.MyID <= 12
select new { o.MyID, o.MyName, o.MyAddress };
grdData.DataSource = Select2;
grdData.DataBind();
}
protected void btnDelete_Click(object sender, EventArgs e)
{
var delete = db.spMyTableDelete(3);
}
protected void btnSelectMulti_Click(object sender, EventArgs e)
{
IMultipleResults results = new MyDataContext().spMyTableSelectMulti();
List<clsMultiRecords.spMyTableSelectMultiResult> result1 = results.GetResult<clsMultiRecords.spMyTableSelectMultiResult>().ToList();
List<clsMultiRecords.spMyTableSelectMultiResult2> result2 = results.GetResult<clsMultiRecords.spMyTableSelectMultiResult2>().ToList();
grdData.DataSource = result2;
grdData.DataBind();
}
protected void btnSelectMultiWithOutputParam_Click(object sender, EventArgs e)
{
string strCustName = "";
var q2 = db.spMyTableSelectMultiWithOutputParam(1, ref strCustName);
Response.Write(strCustName.ToString());
grdData.DataSource = q2;
grdData.DataBind();
}
}
MyDataContext class
#pragma warning disable 1591
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by a tool.
// Runtime Version:2.0.50727.3053
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.Linq;
using System.Data.Linq.Mapping;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
[System.Data.Linq.Mapping.DatabaseAttribute(Name="Northwind")]
public partial class MyDataContext : System.Data.Linq.DataContext
{
private static System.Data.Linq.Mapping.MappingSource mappingSource = new AttributeMappingSource();
#region Extensibility Method Definitions
partial void OnCreated();
#endregion
public MyDataContext() :
base(global::System.Configuration.ConfigurationManager.ConnectionStrings["NorthwindConnectionString"].ConnectionString, mappingSource)
{
OnCreated();
}
public MyDataContext(string connection) :
base(connection, mappingSource)
{
OnCreated();
}
public MyDataContext(System.Data.IDbConnection connection) :
base(connection, mappingSource)
{
OnCreated();
}
public MyDataContext(string connection, System.Data.Linq.Mapping.MappingSource mappingSource) :
base(connection, mappingSource)
{
OnCreated();
}
public MyDataContext(System.Data.IDbConnection connection, System.Data.Linq.Mapping.MappingSource mappingSource) :
base(connection, mappingSource)
{
OnCreated();
}
[Function(Name="dbo.spMyTableInsert")]
public int spMyTableInsert([Parameter(Name="MyName", DbType="VarChar(50)")] string myName, [Parameter(Name="MyAddress", DbType="VarChar(50)")] string myAddress)
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())), myName, myAddress);
return ((int)(result.ReturnValue));
}
[Function(Name="dbo.spMyTableUpdate")]
public int spMyTableUpdate([Parameter(Name="MyID", DbType="Int")] System.Nullable<int> myID, [Parameter(Name="MyName", DbType="VarChar(50)")] string myName, [Parameter(Name="MyAddress", DbType="VarChar(50)")] string myAddress)
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())), myID, myName, myAddress);
return ((int)(result.ReturnValue));
}
[Function(Name="dbo.spMyTableSelect")]
public ISingleResult<spMyTableSelectResult> spMyTableSelect()
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
return ((ISingleResult<spMyTableSelectResult>)(result.ReturnValue));
}
[Function(Name="dbo.spMyTableDelete")]
public int spMyTableDelete([Parameter(Name="MyID", DbType="Int")] System.Nullable<int> myID)
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())), myID);
return ((int)(result.ReturnValue));
}
//[Function(Name = "dbo.spMyTableSelectMulti")]
//public ISingleResult<spMyTableSelectMultiResult> spMyTableSelectMulti()
//{
// IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
// return ((ISingleResult<spMyTableSelectMultiResult>)(result.ReturnValue));
//}
[Function(Name = "dbo.spMyTableSelectMulti"), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult)), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult2))]
public IMultipleResults spMyTableSelectMulti()
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
return ((IMultipleResults)(result.ReturnValue));
}
//[Function(Name = "dbo.spMyTableSelectMulti")]
//public IMultipleResults spMyTableSelectMulti()
//{
// IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
// return (IMultipleResults)(result.ReturnValue);
//}
[Function(Name="dbo.spMyTableSelectMultiWithOutputParam")]
public ISingleResult<spMyTableSelectMultiWithOutputParamResult> spMyTableSelectMultiWithOutputParam([Parameter(Name="MyID", DbType="Int")] System.Nullable<int> myID, [Parameter(Name="MyName", DbType="VarChar(50)")] ref string myName)
{
IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())), myID, myName);
myName = ((string)(result.GetParameterValue(1)));
return ((ISingleResult<spMyTableSelectMultiWithOutputParamResult>)(result.ReturnValue));
}
}
public partial class spMyTableSelectResult
{
private int _MyID;
private string _MyName;
private string _MyAddress;
public spMyTableSelectResult()
{
}
[Column(Storage="_MyID", DbType="Int NOT NULL")]
public int MyID
{
get
{
return this._MyID;
}
set
{
if ((this._MyID != value))
{
this._MyID = value;
}
}
}
[Column(Storage="_MyName", DbType="VarChar(50)")]
public string MyName
{
get
{
return this._MyName;
}
set
{
if ((this._MyName != value))
{
this._MyName = value;
}
}
}
[Column(Storage="_MyAddress", DbType="VarChar(50)")]
public string MyAddress
{
get
{
return this._MyAddress;
}
set
{
if ((this._MyAddress != value))
{
this._MyAddress = value;
}
}
}
}
//public partial class spMyTableSelectMultiResult
//{
// private int _MyID;
// private string _MyName;
// private string _MyAddress;
// public spMyTableSelectMultiResult()
// {
// }
// [Column(Storage="_MyID", DbType="Int NOT NULL")]
// public int MyID
// {
// get
// {
// return this._MyID;
// }
// set
// {
// if ((this._MyID != value))
// {
// this._MyID = value;
// }
// }
// }
// [Column(Storage="_MyName", DbType="VarChar(50)")]
// public string MyName
// {
// get
// {
// return this._MyName;
// }
// set
// {
// if ((this._MyName != value))
// {
// this._MyName = value;
// }
// }
// }
// [Column(Storage="_MyAddress", DbType="VarChar(50)")]
// public string MyAddress
// {
// get
// {
// return this._MyAddress;
// }
// set
// {
// if ((this._MyAddress != value))
// {
// this._MyAddress = value;
// }
// }
// }
//}
public partial class spMyTableSelectMultiWithOutputParamResult
{
private int _MyID;
private string _MyName;
private string _MyAddress;
public spMyTableSelectMultiWithOutputParamResult()
{
}
[Column(Storage="_MyID", DbType="Int NOT NULL")]
public int MyID
{
get
{
return this._MyID;
}
set
{
if ((this._MyID != value))
{
this._MyID = value;
}
}
}
[Column(Storage="_MyName", DbType="VarChar(50)")]
public string MyName
{
get
{
return this._MyName;
}
set
{
if ((this._MyName != value))
{
this._MyName = value;
}
}
}
[Column(Storage="_MyAddress", DbType="VarChar(50)")]
public string MyAddress
{
get
{
return this._MyAddress;
}
set
{
if ((this._MyAddress != value))
{
this._MyAddress = value;
}
}
}
}
#pragma warning restore 1591
clsMultiRecords.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.Linq;
using System.Data.Linq.Mapping;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
/// <summary>
/// Summary description for clsMultiRecords
/// </summary>
public class clsMultiRecords
{
public clsMultiRecords()
{
//
// TODO: Add constructor logic here
//
}
//[Function(Name = "dbo.spMyTableSelectMulti"), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult)), ResultType(typeof(clsMultiRecords.spMyTableSelectMultiResult2))]
//public IMultipleResults spMyTableSelectMulti()
//{
// IExecuteResult result = this.ExecuteMethodCall(this, ((MethodInfo)(MethodInfo.GetCurrentMethod())));
// return ((IMultipleResults)(result.ReturnValue));
//}
public partial class spMyTableSelectMultiResult
{
private int _MyID;
private string _MyName;
private string _MyAddress;
public spMyTableSelectMultiResult()
{
}
[Column(Storage = "_MyID", DbType = "Int NOT NULL")]
public int MyID
{
get
{
return this._MyID;
}
set
{
if ((this._MyID != value))
{
this._MyID = value;
}
}
}
[Column(Storage = "_MyName", DbType = "VarChar(50)")]
public string MyName
{
get
{
return this._MyName;
}
set
{
if ((this._MyName != value))
{
this._MyName = value;
}
}
}
[Column(Storage = "_MyAddress", DbType = "VarChar(50)")]
public string MyAddress
{
get
{
return this._MyAddress;
}
set
{
if ((this._MyAddress != value))
{
this._MyAddress = value;
}
}
}
}
public partial class spMyTableSelectMultiResult2
{
private int _MyID;
private string _MyName;
private string _MyAddress;
public spMyTableSelectMultiResult2()
{
}
[Column(Storage = "_MyID", DbType = "Int NOT NULL")]
public int MyID
{
get
{
return this._MyID;
}
set
{
if ((this._MyID != value))
{
this._MyID = value;
}
}
}
[Column(Storage = "_MyName", DbType = "VarChar(50)")]
public string MyName
{
get
{
return this._MyName;
}
set
{
if ((this._MyName != value))
{
this._MyName = value;
}
}
}
[Column(Storage = "_MyAddress", DbType = "VarChar(50)")]
public string MyAddress
{
get
{
return this._MyAddress;
}
set
{
if ((this._MyAddress != value))
{
this._MyAddress = value;
}
}
}
}
}